@oid said:
@ddw_music Long sysex messages work just fine if you treat them as what they are and what pd is quite good at dealing with, streams.
I do understand what you're saying, and I began with the same assumption -- that [midiout] should be just a low-level conduit -- byte goes in, byte gets sent out.
Unfortunately it doesn't work that way in practice.
My test scenario looks like this. Launch SuperCollider and Pure Data. It helps in my case that I'm testing in Linux, where I can create and remove MIDI connections arbitrarily in qjackctl's ALSA tab.
SC code:
MIDIClient.init;
MIDIIn.connect(0, MIDIClient.sources.detectIndex { |src| src.device.containsi("pure") });
m = MIDIOut(0).connect(MIDIClient.destinations.detectIndex { |dst| dst.device.containsi("pure") });
m.connect(MIDIClient.destinations.detectIndex { |dst| dst.device.containsi("superc") });
MIDIdef.sysex(\a, { |... args| args.postln });
Then I need to go to qjackctl and connect Pd's MIDI output to Pd's MIDI input.
At this point, I can send MIDI from either Pd or SuperCollider, and both SC and Pd will respond.
Pd patch, implementing "stream of bytes" (btw I tested both [sysexin] and [midiin], no difference in the results):
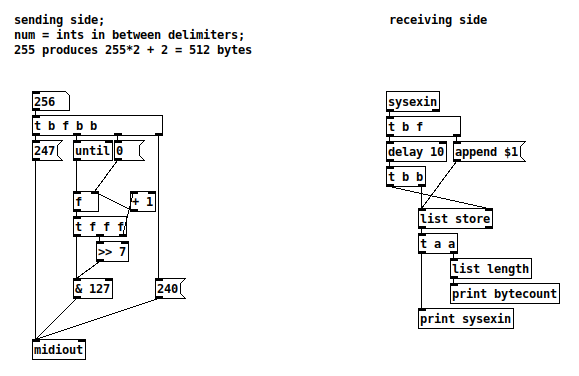
sysex-stream.pd
(Note also: Because I am collecting the MIDI-in bytes into a list, I also checked whether there is a maximum list length. There may be, but we won't hit any such limit in these tests -- I could go up to 5000 numbers in a list, no problem. So, in case of any message truncation, it must be happening in the MIDI objects, not in list handling.)
The test case will be to send a packet of n 14-bit integers (MSB first). The total packet size will be n*2 + 2
, so if we want to produce exactly b
bytes, n = (b / 2) - 1
.
SuperCollider sending:
(
f = { |n|
var out = Int8Array[240];
n.do { |i|
out = out.add(i >> 7 & 127).add(i & 127);
};
out.add(247);
};
)
// small packet
m.sysex(f.value(10)); // SC OK, Pd OK (22 bytes)
// 512 bytes
m.sysex(f.value(255)); // both OK
// 514 bytes
m.sysex(f.value(256)); // SC OK, Pd did not print
And my results for Pd sending:
- 22 bytes (n = 10): Both OK
- 512 bytes (n = 255): Both OK
- 514 bytes (n = 256): SC did not respond. Pd printed only 512 bytes (truncated).
Conclusions:
- When SC sent 514 bytes, SC received 514 bytes, so we know SC is sending a complete packet.
- Pd did not respond, suggesting that both [sysexin] and [midiin] simply stopped listening after 512 bytes, did not process the ending delimiter, and thus did not output the packet at all.
- When Pd was asked to send 514 bytes, Pd received only 512 bytes. This suggests that [midiout] is buffering the incoming bytes, waiting for a complete packet before sending, but it must have a limit of 512 bytes, causing the message to be truncated. (And SC didn't respond at all, which suggests that the closing delimiter was never sent.)
So again... in principle, you're correct -- it's technically possible for some [midiout] object to relay the data out, byte by byte. But neither [midiin] nor [midiout] are implemented that way.
hjh